10 JavaScript topics that every programmer should know.
Hello, everyone today I am going to show you 10 javascript interesting topics that every programmer should know. Are you ready for it? so, without doing any further do let’s get started.
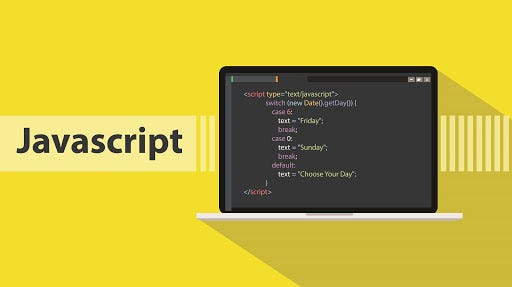
charAt() function
When we want to find a character into a string by using index then we need to use the charAt() function. For example:
const name = 'javascript'
name.charAt(3) // expected output 'a'
concat() function
Sometimes we need to combine two strings. To combine two or more strings we can use the concat() function. You will understand it better by seeing the example below:
const firstName = 'Lional';
const lastName = 'Messi';
const fullName = firstName.concat(lastName); // expected output: 'LionelMessi'
Oh, This is not the result that we want. so, how can we solve it. Wait I am showing you the correct one.
const firstName = 'Lional';
const lastName = 'Messi';
const fullName = firstName.concat(' ', lastName); // expected output: 'Lionel Messi'
We can use an additional empty string to give a space between two names.
The slice() method
The slice() method returns a new string of the existing string. When we need some specific part of a string then slice() method helps us to easily cut the specific part. here is an example:
const speech = 'now i am learning javascript string slice method';
const newSpeech = speech.slice(3, 29);
// expected output: 'i am learning javascript'
The difference between double equal(==) and Tripple equal(===)
Double equal doesn’t check the data type when we compare something. on the other hand, Tripple equals check the data type. When its data type is matched it will return true, if it does not match it will return false. see the example below:
const compare1 = (1256 == '1256');
console.log(compare1); // true
const compare2 = (1256 === '1256');
console.log(compare2); // false
Add an item to the last of an array
There is a simple way to push an item to the last of an array. see the example below you will understand it clearly:
const friends = ['saurav', 'sumon', 'rakib', 'anik'];
friends.push('billa');
console.log(friends);
// expected output friends = ['saurav', 'sumon', 'rakib', 'anik', 'billa'];
Add an item to the first of an array
There is a simple way to push an item to the first of an array. see the example below you will understand it clearly:
const friends = ['saurav', 'sumon', 'rakib', 'anik'];
friends.unshift('billa');
console.log(friends);
// expected output friends = ['billa', 'saurav', 'sumon', 'rakib', 'anik'];
Generate a random number from 1 to 20
Javascript has a built-in function called Math.random(). By which we can generate a random number. See the example below:
const random = Math.round(Math.random() * 10);
console.log(random); // 1, 2, 3, 4, 5, 6, 7, 8, 9, or 10 any of them
Reverse an array in javascript
Here is the way to reverse an array in javascript. example below:
const array1 = ['one', 'two', 'three'];
console.log('array1:', array1);
// expected output: "array1:" Array ["one", "two", "three"]const reversed = array1.reverse();
console.log('reversed:', reversed);
// expected output: "reversed:" Array ["three", "two", "one"]
javascript array sort
Here is the way to sort an array in javascript. example below:
var num= [4, 36, 3, 8, 45, 7];
num.sort();
console.log(num) // [ 3, 36, 4, 45, 7, 8 ]
Find the max number from an array
Here is the way to sort an array in javascript. example below:
console.log(Math.max(1, 3, 2));
// // expected output: 3
So, guys, this is the end of today. In the future, I will discuss other topics. thank you.